Introduction
The best approach is NOT to use the lib folder. If you need third party libraries, declare them in platformio.ini and PlatformIO will take care of the rest. The lib folder is needed if you want to use a local copy of a third-party library and modify it for your particular project. Collect2.exe: error: ld returned 1 exit status Process terminated with status 1 (0 minute(s), 0 second(s)). Is legal in C. Ignore this post.
In this article I'll be looking at the 'undefined reference' error message (or 'unresolved external symbol, for Visual C++ users). This is not actually a message from the compiler, but is emitted by the linker, so the first thing to do is to understand what the linker is, and what it does.

Linker 101
To understand the linker, you have to understand how C++ programs are built. For all but the very simplest programs, the program is composed of multiple C++ source files (also known as 'translation units'). These are compiled separately, using the C++ compiler, to produce object code files (files with a .o or a .obj extension) which contain machine code. Each object code file knows nothing about the others, so if you call a function from one object file that exists in another, the compiler cannot provide the address of the called function.
This is where the the linker comes in. Once all the object files have been produced, the linker looks at them and works out what the final addresses of functions in the executable will be. It then patches up the addresses the compiler could not provide. It does the same for any libraries (.a and .lib files) you may be using. And finally it writes the executable file out to disk.
The linker is normally a separate program from the compiler (for example, the GCC linker is called ld) but will normally be called for you when you use your compiler suite's driver program (so the GCC driver g++ will call ld for you).
Now you can easily arrange, record and produce your own music on your PC or MAC. The RP350 gives you guitar tones from jazz, blues and rock to full out metal - all recordable directly to PC or Mac via the RP350's USB streaming output. Digitech rp355 patch download. The RP350 guitar multi-effect processor now includes Cubase LE4 music production software. Cubase LE4 is not only a top-notch 48 channel recording DAW, but includes VST instruments like the HALion soft synth completing your recording experience. Now more that ever, nearly unlimited guitar tones are obtainable while other instruments are at your fingertips - it's time to create your hit record!
Traditionally, linker technology has lagged behind compilers, mostly because it's generally more fun to build a compiler than to build a linker. And linkers do not necessarily have access to the source code for the object files they are linking. Put together, you get a situation where linker errors, and the reasons for them, can be cryptic in the extreme.
Undefined reference
Shader model 3.0 gpu driver. I believe from research that it supports shader model 4.1 but as already stated, not DX11 only DX10.1 you will need to buy a dedicated graphics card to run this game or buy a newer cpu with higher DX capability. Gpu Shader 3 0 free download - Photo Story 3 for Windows, Doom 3 demo, The Sims 3 Super Patcher, and many more programs. Free shader model 3.0 free download download software at UpdateStar - Intel X3000 Chipset incorporates key features available in previous Intel Graphics versions like Dynamic Video Memory Technology (DVMT) as well as hardware acceleration for 3D graphics that utilize Microsoft DirectX. 9.0C and OpenGL. 1.5X. Shader Model 3.0 Video Card The 30 vertex shader model (vs30) expands on the features of vs20 with more powerful register indexing, a set of simplified output registers, the ability to sample a texture in a vertex shader, and the ability to control the rate at which shader inputs are initialized. NVIDIA CineFX 3.0 is poised to unleash a new level of programming creativity. With full DirectX 9.0 Shader Model 3.0 support, the newest GeForce GPUs will soon power a new generation of games with unmatched realism, digital worlds with mind-blowing complexity, and lifelike characters that move through cinematic-quality environments.
Roland d 50 vst free download. You may only post music from established artists outside of the weekly pinned threads to ask specific production related questions (e.g.
Put simply, the 'undefined reference' error means you have a reference (nothing to do with the C++ reference type) to a name (function, variable, constant etc.) in your program that the linker cannot find a definition for when it looks through all the object files and libraries that make up your project. There are any number of reasons why it can't find the definition – we'll look at the commonest ones now.
No Definition
Probably the most common reason for unresolved reference errors is that you simply have not defined the thing you are referencing. This code illustrates the problem:
Here, we have a declaration of the function foo(), which we call in main(), but no definition. So we get the error (slightly edited for clarity):
Acer aspire cd dvd driver. Max DVD read speed 8x. Max CD read speed 24x and max CD burn speed 8x.
The way to fix it is to provide the definition:
Wrong Definition
Another common error is to provide a definition that does not match up with declaration (or vice versa). For example, if the code above we had provided a definition of foo() that looked like this:
then we would still get an error from the linker because the signatures (name, plus parameter list types) of the declaration and definition don't match, so the definition actually defines a completely different function from the one in the declaration. To avoid this problem, take some care when writing declarations and definitions, and remember that things like references, pointers and const all count towards making a function signature unique.
Didn't Link Object File
This is another common problem. Suppose you have two C++ source files:
Collect2 Error Ld Returned 1 Exit Status
and:
If you compile f1.cpp on its own you get this:
Collect2 Exe Error Dev C 2b 2b 1b
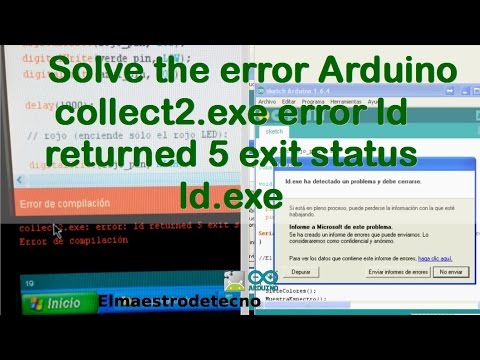
Linker 101
To understand the linker, you have to understand how C++ programs are built. For all but the very simplest programs, the program is composed of multiple C++ source files (also known as 'translation units'). These are compiled separately, using the C++ compiler, to produce object code files (files with a .o or a .obj extension) which contain machine code. Each object code file knows nothing about the others, so if you call a function from one object file that exists in another, the compiler cannot provide the address of the called function.
This is where the the linker comes in. Once all the object files have been produced, the linker looks at them and works out what the final addresses of functions in the executable will be. It then patches up the addresses the compiler could not provide. It does the same for any libraries (.a and .lib files) you may be using. And finally it writes the executable file out to disk.
The linker is normally a separate program from the compiler (for example, the GCC linker is called ld) but will normally be called for you when you use your compiler suite's driver program (so the GCC driver g++ will call ld for you).
Now you can easily arrange, record and produce your own music on your PC or MAC. The RP350 gives you guitar tones from jazz, blues and rock to full out metal - all recordable directly to PC or Mac via the RP350's USB streaming output. Digitech rp355 patch download. The RP350 guitar multi-effect processor now includes Cubase LE4 music production software. Cubase LE4 is not only a top-notch 48 channel recording DAW, but includes VST instruments like the HALion soft synth completing your recording experience. Now more that ever, nearly unlimited guitar tones are obtainable while other instruments are at your fingertips - it's time to create your hit record!
Traditionally, linker technology has lagged behind compilers, mostly because it's generally more fun to build a compiler than to build a linker. And linkers do not necessarily have access to the source code for the object files they are linking. Put together, you get a situation where linker errors, and the reasons for them, can be cryptic in the extreme.
Undefined reference
Shader model 3.0 gpu driver. I believe from research that it supports shader model 4.1 but as already stated, not DX11 only DX10.1 you will need to buy a dedicated graphics card to run this game or buy a newer cpu with higher DX capability. Gpu Shader 3 0 free download - Photo Story 3 for Windows, Doom 3 demo, The Sims 3 Super Patcher, and many more programs. Free shader model 3.0 free download download software at UpdateStar - Intel X3000 Chipset incorporates key features available in previous Intel Graphics versions like Dynamic Video Memory Technology (DVMT) as well as hardware acceleration for 3D graphics that utilize Microsoft DirectX. 9.0C and OpenGL. 1.5X. Shader Model 3.0 Video Card The 30 vertex shader model (vs30) expands on the features of vs20 with more powerful register indexing, a set of simplified output registers, the ability to sample a texture in a vertex shader, and the ability to control the rate at which shader inputs are initialized. NVIDIA CineFX 3.0 is poised to unleash a new level of programming creativity. With full DirectX 9.0 Shader Model 3.0 support, the newest GeForce GPUs will soon power a new generation of games with unmatched realism, digital worlds with mind-blowing complexity, and lifelike characters that move through cinematic-quality environments.
Roland d 50 vst free download. You may only post music from established artists outside of the weekly pinned threads to ask specific production related questions (e.g.
Put simply, the 'undefined reference' error means you have a reference (nothing to do with the C++ reference type) to a name (function, variable, constant etc.) in your program that the linker cannot find a definition for when it looks through all the object files and libraries that make up your project. There are any number of reasons why it can't find the definition – we'll look at the commonest ones now.
No Definition
Probably the most common reason for unresolved reference errors is that you simply have not defined the thing you are referencing. This code illustrates the problem:
Here, we have a declaration of the function foo(), which we call in main(), but no definition. So we get the error (slightly edited for clarity):
Acer aspire cd dvd driver. Max DVD read speed 8x. Max CD read speed 24x and max CD burn speed 8x.
The way to fix it is to provide the definition:
Wrong Definition
Another common error is to provide a definition that does not match up with declaration (or vice versa). For example, if the code above we had provided a definition of foo() that looked like this:
then we would still get an error from the linker because the signatures (name, plus parameter list types) of the declaration and definition don't match, so the definition actually defines a completely different function from the one in the declaration. To avoid this problem, take some care when writing declarations and definitions, and remember that things like references, pointers and const all count towards making a function signature unique.
Didn't Link Object File
This is another common problem. Suppose you have two C++ source files:
Collect2 Error Ld Returned 1 Exit Status
and:
If you compile f1.cpp on its own you get this:
Collect2 Exe Error Dev C 2b 2b 1b
and if you compile f2.cpp on its own, you get this even more frightening one:
In this situation, you need to compile both the the source files on the same command line, for example, using GCC:
or if you have compiled them separately down to object files:
For further information on compiling and linking multiple files in C++, particularly with GCC, please see my series of three blog articles starting here.
Wrong Project Type
The linker error regarding WinMain above can occur in a number of situations, particularly when you are using a C++ IDE such as CodeBlocks or Visual Studio. These IDEs offer you a number of project types such as 'Windows Application' and 'Console Application'. If you want to write a program that has a int main() function in it, always make sure that you choose 'Console Application', otherwise the IDE may configure the linker to expect to find a WinMain() function instead.
No Library
To understand this issue, remember that a header file (.h) is not a library. The linker neither knows nor cares about header files – it cares about .a and .lib files. So if you get a linker error regarding a name that is in a library you are using, it is almost certainly because you have not linked with that library. To perform the linkage, if you are using an IDE you can normally simply add the library to your project, if using the command line, once again please see my series of blog articles on the GCC command line starting here, which describes some other linker issues you may have.
Collect2 Error Ld
Conclusion
Football Manager 2013 celebrates 20 years of games from the people at Sports Interactive by introducing an array of new features. Free football manager 2013 crack download. Football Manager is the best-selling, most realistic football management series ever made. This year's version allows you to take control of any club in more than 50 nations across the world and includes all of Europe's biggest leagues as well as database of over 500,000 real-world players and staff. As well as some landmark new features in the Career Mode, there are now new ways to enjoy your Football Manager experience.
The unresolved reference error can have many causes, far from all of which have been described here. But it's not magic – like all errors it means that you have done something wrong, in you code and/or your project's configuration, and you need to take some time to sit down, think logically, and figure out what.